The motivation behind this page is to provide a very broad overview of what are usually present or feed into successful product delivery work.
This list is definitely not prescriptive, other things can and should be added or removed to fit one’s environment and circumstances.
The very basics of software development, object-oriented programming, design patterns or hygienne factors of a technical team or prodcut team are not covered, as the expectation is that the team are already aware of these and other standard industry practices.
PS: this list is not the silver bullet!
Before Starting
Bake-in quality and security into your products
You build it, you run it; You break it, you fix it
UX
- Make sure UX is an integral part of your delivery team
- Experiment. Experiment. Experiment
- Prototype. Prototype. Prototype.
- Early customer feedback loops
- Get involved in ideation, user surveys, research and design facilitation
- Involve and experiment with real users
- Your customers and users are also your stakeholders
- Improve and grow your Design Literacy
- Collect and use data to gauge whether you’ve hit the mark
Usability makes or breaks your product
Architecture
- Follow the Evolutionary Architecture concept
- Ivory-tower architecture doesn’t work
- Do architecture often and in collaboration
- Remove hard-coupling and dependencies
- OAS based APIs and microservices, consider using Swagger
- Contracts based development for both internal and external components - use mocks and stubs as part of unit testing
- Microservice architecture is not the silver bullet
- Consider other options e.g. serverless, PaaS
- Design for failure
- Think self-monitoring, self-regulating and self-healing mechanisms
- Use the the Technology Radar from Thoughtworks to guide your team’s architecture decisions
“Can’t do architecture without programming, and can’t do programming without architecture” - Martin Fowler
Development environment
- Choose a well-known (and free) IDE
- Documented, easy and quick to set up
- Repeatable, rebuildable everytime - no snowflake setup
- No special tweaks if bit required,use tools out-of-the-box and standardised
- Use automated code checker/linter e.g. SonarLint in Eclipse
- Use automated build tool e.g. Maven or Cake and Fake
Repositories
- Git is preferred - github, gitlab or BitBucket
- Learn git, follow best practices
- Understand how to use git, and understand how distributed repo works
- Implement Versioning policy
- Use Trunk Based Development and Short-Lived Branches strategies
Coding convention
- Conventions for different languages, Google’s Java style
- Conventions should be standardised across the organisation
- Follow the convention and include it in code quality check
- Consistency in the codebase is a must; code individualisation is unacceptable
- Make sure everyone in the team understands and follows the agreed coding convention
Development practices
- Always do peer review through Pull Requests mechanism
- Pair-programming could reduce the need for extensive peer-review
- Do documentation in Readme file in the repo
- Self-documenting code is necessary
- Follow the Boy Scout rules - Clean Code book p14
- No Broken Windows - Clean Code book p8
- Understand what Refactoring is and Just Do It
- Understand what Technical Debt is, and incur when appropriate
- Alignment to architectural and technology governance
“Keep the Codebase Healthy” - Martin Fowler
Security - DevSecOps
- Security engineer needs to be part of the team
- Check for vulnerabilities on own code and all dependencies
- From 12-Factor App:
- follow the 2nd Factor - dependencies
- follow the 3rd Factor - configuration parameters
- Implement OWASP Proactive Controls (the list is ordered in importance)
** Run OWASP ZAP to check your application’s security measures
- Self-service security, and make it part of the build: snyk, Brakeman, dependency checks, plugin for SonarQube
- Docker containers
- Carry out targeted DAST at Acceptance or UAT
- Automated functional and integration testing of security features
- Automated security attacks, using Gauntlt or other tools
- Automated infrastructure security testing e.g. InSpec
- Carry out targeted manual Penetration Testing
Quality
- TDD - you need to understand this
- Automate. Automate. Automate
- Follow the principles of Testing Pyramid
- Beware of the Testing Cupcake
- Determine the layers of the pyramid for your application - start simple
- Manual/exploratory testing (the cloud on top of the pyramid) will need to be kept to the minimum, and as necessary.
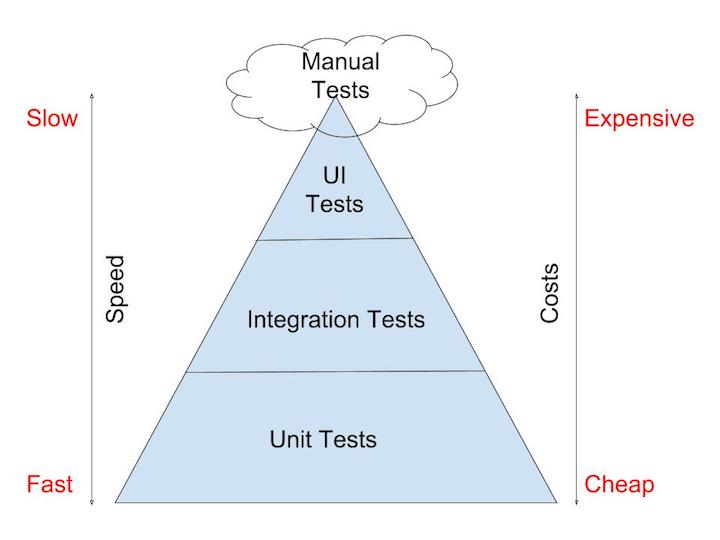
- For automated tests
- Run UI tests using Chrome’s headless mode
- Only do the necessary validation with acceptance tests, everything else covered by unit tests and a smaller layer of integration tests.
- “Record and playback testing” is not automated testing will create non-deterministic tests.
- Understand Stubbing and Mocking
- BDD should be used “in all the places where the business has reason to have opinions about the behaviour.”
- For API testing consider using automated contract testing tool such as Pact
- Understand what Test Coverage is, and determine the right coverage as part of your static code analysis
- Never have non-deterministic test
- Test based on Risk Assessment
- Do load and performance testing
- Do security and penetration tests
- Automate. Automate. Automate
Static code analysis
- Use SonarQube or similar tools
** SonarQube’s plug-in for checking on software licenses
- Include code convention as part of the check
- Agree on the metrics your team should start with
- Monitored and adjusted as your team matures
- Include this in the CI pipeline, and fail the build when your quality drops
Principles of CI/CD
Continuous Integration
- Automated build and notifications
- When a build should be activated, at every commit?
- Build should stop at ANY test failure - no excuse
- Build should stop at a predetermined decrease of code quality - use SonarQube or similar tool to check
- Roll your own, Jenkins, Hudson, Octopus etc.
- SaaS: Codeship, Travis CI, Bamboo, Hudson, GoCD
Continuous Delivery/Deployment
- Infrastructure as Code, include unit tests!
- Automatically build the release package
- Automatically deploy to intended environments
- Treat servers as cattle, not pets
- Release package will be promoted to the next stage only on successful build
- Consistent server build and environment, no snowflake or pets server or environment
- 10th Factor - all environments should be very similar, dev, test, UAT, QA, prod.
- Utilise containers i.e. Docker
- Utilise an orchestration manager e.g. Kubernetes, Docker Swarm, Apache Mesos
- Utilise a configuration manager, Infrastructure as Code, e.g. Ansible, Chef, Puppet, even on PaaS
- Consider the right deployment technique:
- A/B testing
- Red/Blue deployment
- Canary
- Feature toggles
- Dark launches
- Decouple deployment from release
A deployment pipeline is, in essence, an automated implementation of your application’s build, deploy, test, and release process. - Continuous Delivery book
Data(base)
- Should have a strategy to optimise data usage and storage
- Evolutionary database design
- Data obsfucation or masking must be part of the workflow to protect private and sensitive data
- Build in metrics to provide data to the business and for health monitoring
- Consider support scenario
- Be Data Informed - Use data to help your team make decisions
- Use data to make your life easier
Support - Monitoring
- Consider the principle of Least Privilege for access control
- Consider support requirements
- Put monitoring everywhere, prioritise alerting on component’s importance, resiliency, set-up, backup etc.
- New Relic, OMS, Splunk, ELK, Raygun
- Build a dashboard which will be useful for all support levels, consider Splunk, Grafana
Support - Alerting
- Consider the application’s SLA
- Consider the support agreements
- Consider Segregation of Duties
- Determine the metrics that will trigger alerts
- Alert types e.g. Info, Warning etc.
- Select the appropriate tools to make your life easy: IM, SMS, email, flashing lights, sirens etc.
Resources